What Is FlyWeb?
FlyWeb is a project at Mozilla focused on bringing a new set of APIs to the browser for advertising and discovering local-area web servers.
Getting Started
Setting up Firefox Developer Edition (Aurora)
-
Install Firefox Developer Edition for Desktop or Firefox Aurora for Android
-
Navigate to
about:config
and toggle ondom.flyweb.enabled
IMPORTANT To ensure safety, you should NOT enable this flag when using your everyday Firefox profile. This technology is still in a very early experimental state and although the source code has passed an initial security review, it is still possible that security vulnerabilities exist in this feature.
-
On Desktop, select Customize from the
menu and drag the
icon to any toolbar
On Android, tap FlyWeb from the
menu or enter
about:flyweb
in the address bar
Publishing a server from a web page
The easiest way to get started building FlyWeb-enabled apps is via the FlyWeb Web API. This allows ordinary web pages to start local web servers and advertise them as FlyWeb services to nearby browsers.
navigator.publishServer('Hello FlyWeb').then(function(server) {
server.onfetch = function(event) {
var html = '<h1>Hello FlyWeb!</h1>' +
'<h3>You requested: ' + event.request.url + '</h3>';
event.respondWith(new Response(html, {
headers: { 'Content-Type': 'text/html' }
}));
};
});
In the example above, a FlyWeb service will be advertised called "Hello FlyWeb" that will respond with a simple HTML page that echoes the URL requested by the connected client.
Building a FlyWeb service on a Raspberry Pi
Because FlyWeb services are just local web servers, building a FlyWeb-enabled device with a Raspberry Pi is easy. Since Node.js can run on a Raspberry Pi, its possible to leverage the existing Node.js ecosystem for building server-side web applications including frameworks such as Express. The only additional functionality required to make an ordinary Node.js web server a FlyWeb service is a proper mDNS advertisement. Fortunately, an NPM module called mdns already exists for handling this important detail.
var http = require('http');
var mdns = require('mdns');
var port = parseInt(process.env.PORT || '3000');
var server = http.createServer(function(request, response) {
var html = '<h1>Hello FlyWeb from Node JS!</h1>' +
'<h3>You requested: ' + request.url + '</h3>';
response.writeHead(200, { 'Content-Type': 'text/html' });
response.end(html);
});
var advertisement = mdns.createAdvertisement(mdns.tcp('flyweb'), port, {
name: 'Hello Node FlyWeb'
});
server.listen(port, function() {
console.log('Server listening on port ' + port);
advertisement.start();
});
This example can be run in Node.js on a Raspberry Pi (or a laptop computer) and will advertise a FlyWeb service called "Hello Node FlyWeb" that can be discovered by clients on the local network running Firefox with FlyWeb enabled.
Showcase
Take FlyWeb for a test drive with these example apps and demos
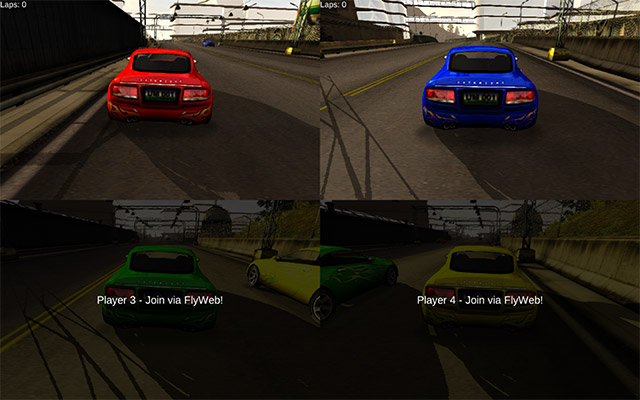
FlyWeb GP
A demonstration of a Unity 3D WebGL racing game that allows players to join in and control the game with their web browser
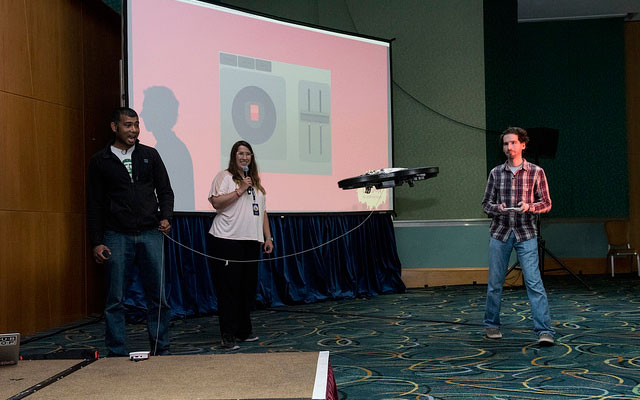
FlyWeb Quadcopter
Control an AR.Drone quadcopter with your browser using a simple Node.js server that can run on a Raspberry Pi
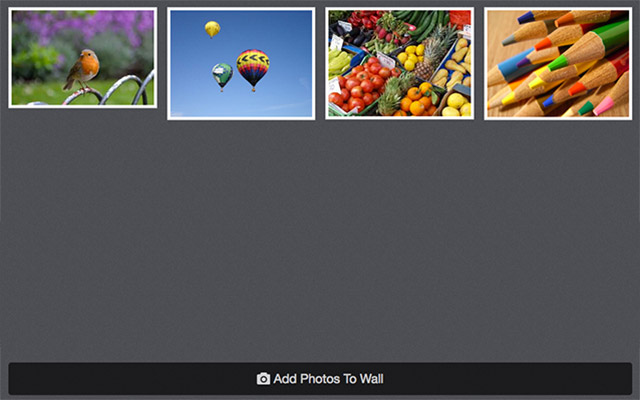
Photo Wall
A simple photo-sharing app that lets nearby users join via FlyWeb to view photos on a wall and share their own in real-time
Community
We are excited to hear about your ideas for using FlyWeb in new and unique ways! Please drop us a line in any of these channels if you have any questions or need any help getting your projects off the ground.
- #flyweb on IRC @ irc.mozilla.org (View Log)
- mozflyweb.slack.com on Slack (Sign Up)
- dev-flyweb mailing list on Google Groups